Example 1 – for loop
This is an example of a for loop in python (file Example1). The for loop repeats ten times the two indented lines under the colon.
for n in range(0,10):
print(n)
print(‘Today is a beautiful day’)
print(‘Program finished!’)
This is the program output:
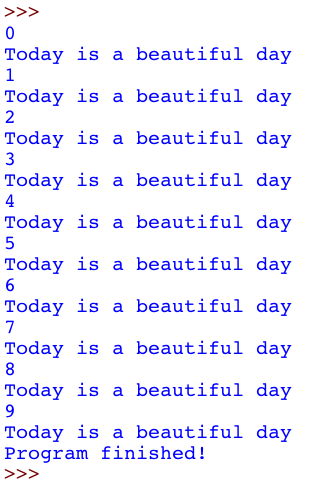
Example 2 – while loop
This is an example of a while loop in Python (file Example2). The while loop repeats ten times the three indented lines under the colon.
x = 0
while x < 10:
print(x)
print(‘Today is a beautiful day’)
x = x + 1
print(‘Program finished!’)
The output is identical to the output of the previous example:
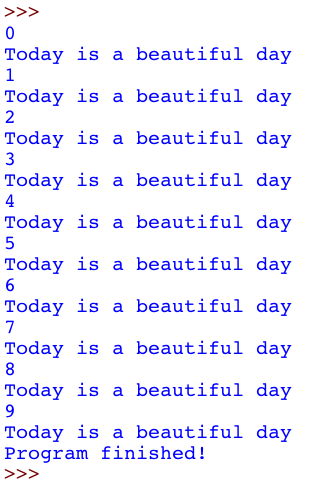
Example 3 – infinite loop with while
This example shows an infinite loop (file Example3). The program keeps repeating the two indented lines under the colon forever, or until the program is interrupted by pressing CTRL+C. The third print statement never gets executed, because the program never gets out from the while loop.
while True:
print(‘Today is a beautiful day’)
print(‘… and yesterday was too!’)
print(‘This line will never be printed!’) # This line will never be printed
# because the program never get out
# from the while loop
Below is the output. The program is interrupted pressing CTRL+C.
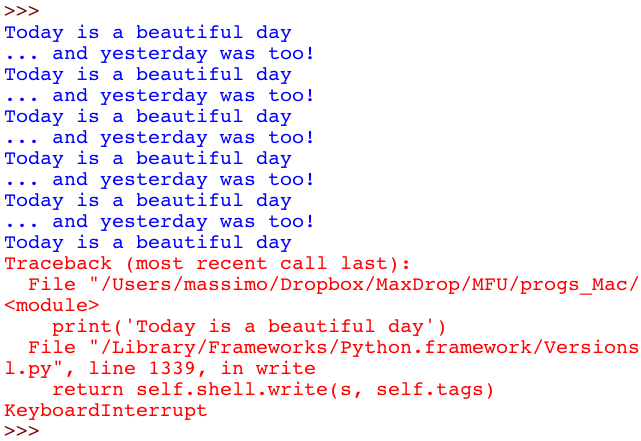